In the realm of graphics programming, Vulkan stands out as a revolutionary API (Application Programming Interface) that offers high-efficiency, cross-platform access to modern GPUs (Graphics Processing Units) used in a wide range of devices from PCs and consoles to mobile devices. Developed by the Khronos Group, Vulkan is designed to provide better performance and more balanced CPU/GPU usage compared to its predecessors and competitors, such as OpenGL and DirectX. This blog post aims to serve as a comprehensive tutorial for beginners interested in learning about Vulkan, covering its core concepts, setup, and a simple rendering example.
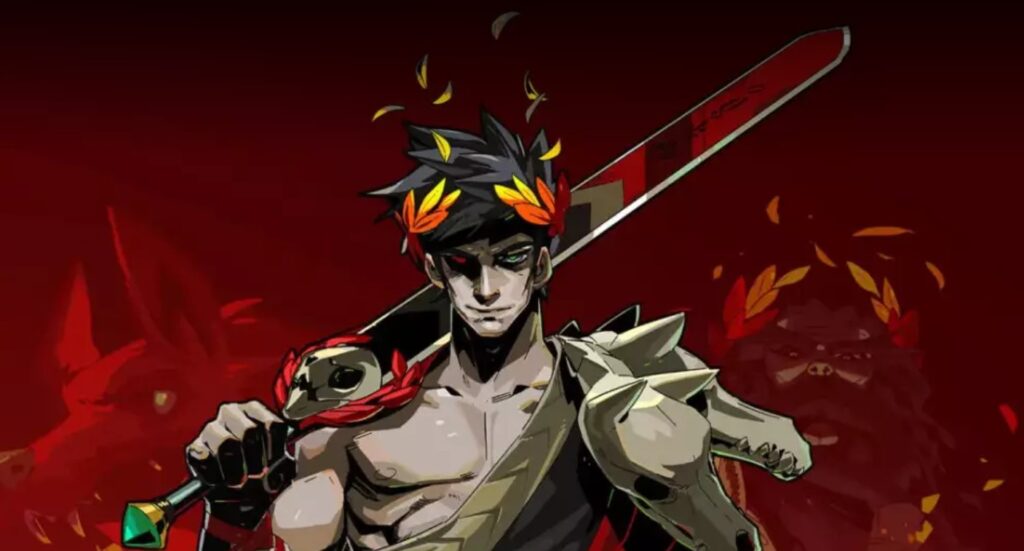
Why Vulkan?
Vulkan’s main advantage lies in its explicit design. Unlike OpenGL, Vulkan requires developers to provide more details about their graphics and compute operations. This upfront work can be more complex and requires a deeper understanding of graphics programming, but it offers several benefits:
- Performance and Efficiency: By giving developers more control over GPU operations, Vulkan can reduce overhead and improve graphics rendering and compute performance.
- Cross-Platform Compatibility: Vulkan runs on multiple platforms, including Windows, Linux, Android, and others, making it easier to develop applications that can run across various devices.
- Fine-Grained Control: Developers have more direct control over memory management, synchronization, and the execution of commands, allowing for sophisticated optimization techniques.
Getting Started with Vulkan
Prerequisites
Before diving into Vulkan, it’s essential to have a basic understanding of graphics programming and familiarity with C or C++ as Vulkan’s API is exposed through these languages.
Installation and Setup
- Vulkan SDK: The first step is to download and install the Vulkan Software Development Kit (SDK) from the official LunarG website. The SDK includes libraries, examples, and essential tools needed to develop with Vulkan.
- Development Environment: Ensure that your development environment, whether it’s Visual Studio, GCC, Clang, or another, is set up correctly to use the Vulkan SDK. This typically involves configuring include directories, library paths, and linker settings.
- Graphics Driver: Make sure your GPU’s graphics driver supports Vulkan. Most modern graphics cards from NVIDIA, AMD, and Intel provide Vulkan support.
Creating a Simple Vulkan Application
Developing a simple application with Vulkan involves several steps, each requiring a careful setup due to Vulkan’s explicit and verbose nature. Here’s a high-level overview:
1. Instance Creation
The first step in any Vulkan application is to create a Vulkan instance. The instance is the connection between your application and the Vulkan library, and it’s created by specifying application details and the desired Vulkan extensions and validation layers.
VkApplicationInfo appInfo = {};
appInfo.sType = VK_STRUCTURE_TYPE_APPLICATION_INFO;
appInfo.pApplicationName = "Hello Vulkan";
appInfo.applicationVersion = VK_MAKE_VERSION(1, 0, 0);
appInfo.pEngineName = "No Engine";
appInfo.engineVersion = VK_MAKE_VERSION(1, 0, 0);
appInfo.apiVersion = VK_API_VERSION_1_0;
VkInstanceCreateInfo createInfo = {};
createInfo.sType = VK_STRUCTURE_TYPE_INSTANCE_CREATE_INFO;
createInfo.pApplicationInfo = &appInfo;
if (vkCreateInstance(&createInfo, nullptr, &instance) != VK_SUCCESS) {
throw std::runtime_error("failed to create instance!");
}
2. Physical and Logical Device Selection
After creating an instance, you need to select a physical device (GPU) and create a logical device to interact with it. This step involves querying the GPU properties and features, then setting up queues and extensions that your application will use.
3. Creating a Window Surface
Vulkan itself does not handle window creation or input events; this must be done using a windowing system like GLFW or SDL. Once the window is created, you need to create a Vulkan surface for the window, allowing Vulkan to interface with it.
4. Graphics Pipeline Setup
The graphics pipeline is the heart of rendering with Vulkan, defining how to process vertices and fragments, along with specifying states like the viewport, rasterizer, shaders, etc. Setting up a pipeline involves creating shader modules, setting pipeline states, and compiling it into a usable form.
5. Drawing Frame
To draw a frame, you need to record commands into a command buffer, telling Vulkan what to draw and how to draw it. This typically involves binding the graphics pipeline, drawing commands, and managing synchronization primitives to ensure proper execution order.
Conclusion
While Vulkan’s steep learning curve can be intimidating, its design allows for unparalleled control and efficiency in graphics applications. This tutorial has only scratched the surface of what’s possible with Vulkan. For those willing to invest the time to master its complexities, Vulkan opens up a world of high-performance, cross-platform graphics programming. Further exploration through official documentation, sample code, and community resources is highly recommended to deepen your understanding and skills in Vulkan development.
Leave a Reply